Analyses Visualization
Table of contents
Classification Example
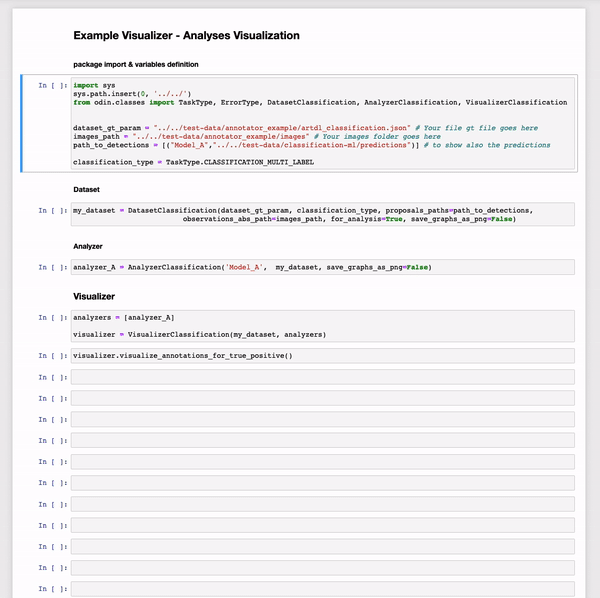
Localization Example
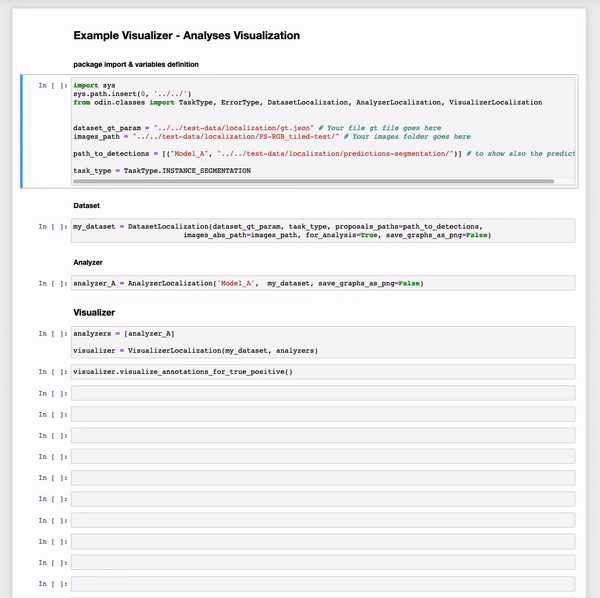
visualize_annotations_for_true_positive()
It shows the ground truth and the predictions based on the true positive analysis.
Parameters
- categories
list, optional- List of categories to be included in the visualization. If not specified, all the categories are included.
(default is None) - model
str, optional- Name of the model used for the analysis. If not specified, it is considered the first provided.
(default is None)
Example
Classification
from odin.classes import VisualizerClassification
my_visualizer = VisualizerClassification(models)
my_visualizer.visualize_annotations_for_true_positive()
Localization
from odin.classes import VisualizerLocalization
my_visualizer = VisualizerLocalization(models)
my_visualizer.visualize_annotations_for_true_positive()
Tasks supported
Binary Classification | Single-label Classification | Multi-label Classification | Object Detection | Instance Segmentation |
---|---|---|---|---|
yes | yes | yes | yes | yes |
visualize_annotations_for_false_positive()
It shows the ground truth and the predictions based on the false positive analysis.
Parameters
- categories
list, optional- List of categories to be included in the visualization. If not specified, all the categories are included.
(default is None) - model
str, optional- Name of the model used for the analysis. If not specified, it is considered the first provided.
(default is None)
Example
Classification
from odin.classes import VisualizerClassification
my_visualizer = VisualizerClassification(models)
my_visualizer.visualize_annotations_for_false_positive()
Localization
from odin.classes import VisualizerLocalization
my_visualizer = VisualizerLocalization(models)
my_visualizer.visualize_annotations_for_false_positive()
Tasks supported
Binary Classification | Single-label Classification | Multi-label Classification | Object Detection | Instance Segmentation |
---|---|---|---|---|
yes | yes | yes | yes | yes |
visualize_annotations_for_false_negative()
It shows the ground truth and the predictions based on the false negative analysis.
Parameters
- categories
list, optional- List of categories to be included in the visualization. If not specified, all the categories are included.
(default is None) - model
str, optional- Name of the model used for the analysis. If not specified, it is considered the first provided.
(default is None)
Example
Classification
from odin.classes import VisualizerClassification
my_visualizer = VisualizerClassification(models)
my_visualizer.visualize_annotations_for_false_negative()
Localization
from odin.classes import VisualizerLocalization
my_visualizer = VisualizerLocalization(models)
my_visualizer.visualize_annotations_for_false_negative()
Tasks supported
Binary Classification | Single-label Classification | Multi-label Classification | Object Detection | Instance Segmentation |
---|---|---|---|---|
yes | yes | yes | yes | yes |
visualize_annotations_for_true_negative()
It shows the ground truth and the predictions based on the true negative analysis.
Parameters
- model
str, optional- Name of the model used for the analysis. If not specified, it is considered the first provided.
(default is None)
Example
Classification
from odin.classes import VisualizerClassification
my_visualizer = VisualizerClassification(models)
my_visualizer.visualize_annotations_for_true_negative()
Tasks supported
Binary Classification | Single-label Classification | Multi-label Classification | Object Detection | Instance Segmentation |
---|---|---|---|---|
yes | no | no | no | no |
visualize_annotations_for_error_type()
It shows the ground truth and the predictions based on the false positive error type analysis.
Parameters
- error_type
ErrorType- Error type to be included in the analysis. The types of error supported are: ErrorType.BACKGROUND, ErrorType.LOCALIZATION (only for localization tasks), ErrorType.SIMILAR_CLASSES, ErrorType.OTHER.
- categories
list, optional- List of categories to be included in the visualization. If not specified, all the categories are included.
(default is None) - model
str, optional- Name of the model used for the analysis. If not specified, it is considered the first provided.
(default is None)
Example
Classification
from odin.classes import VisualizerClassification
my_visualizer = VisualizerClassification(models)
my_visualizer.visualize_annotations_for_error_type(ErrorType.SIMILAR_CLASSES)
Localization
from odin.classes import VisualizerLocalization
my_visualizer = VisualizerLocalization(models)
my_visualizer.visualize_annotations_for_error_type(ErrorType.LOCALIZATION)
Tasks supported
Binary Classification | Single-label Classification | Multi-label Classification | Object Detection | Instance Segmentation |
---|---|---|---|---|
no | yes | yes | yes | yes |